Steps in getting started
- Download Java
- Download Java SDK
- Get an Integrated Development Environment
- Start a project within the IDE
- Code up "Hello world" and run the code
The entry point to a java application is the main function
It is categorized by its (String[] arg) as a parameter to the function
public class MyMainFunction {
/*Java main function example */
public static void main(String[] args) {
}
}
As you can see the main function is wrapped within a class which is part of the object oriented structure of Java Projects.
The name of the project is therfore "MyMainFunction"
In order to pring to the console. We Use System.out.println.
I know it is long and cumbersome, but this is the way it's done.
public class MyMainFunction {
/* Java main function example */
public static void main(String[] args) {
System.out.println("Hello")
}
}
In this example we are printing out "Hello world" to the console when we run the program.
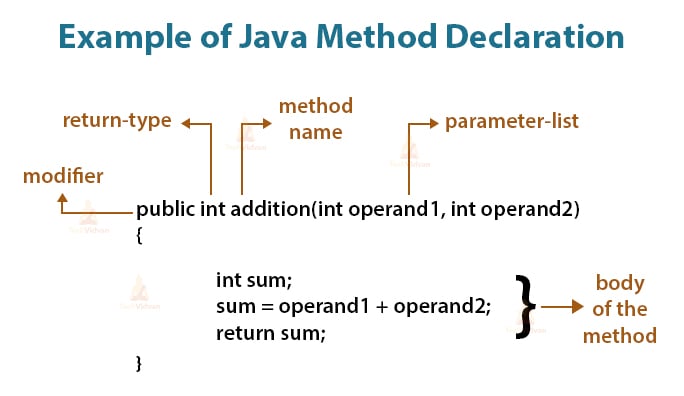
code for copy
Public static void myFunction(String name, int age )
{
//function code
}
Functions are actually called methods in Java. Here is an example of how to declare a Java method.
Java is known as an object orientated programming language
This means that it is easy to represent entities as objects by using classes and encapsulation
An example of this might be a Student class to represent a student
public class Student {
/* Student properties */
private String name;
private int age;
/* Constructor */
public Student(String name, int age){
this.name = name;
this.age = age;
}
/* Getter method */
public String getName() {
return name;
}
/* Setter method */
public void setName(String name) {
this.name = name;
}
We use this class by doing the following:
Student student1 = new Student("Jimmy" , 19);
String jimmyName = student1.getName();
student1.setName("Kevin");
String kevinName = student1.getName();